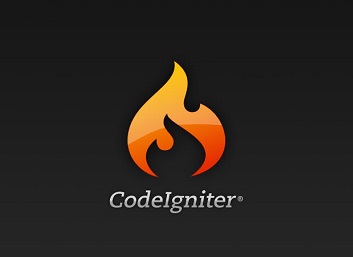
Hướng dẫn tạo form đăng nhập đơn giản trên CoedeIgniter
Bước 1: Tạo cơ sở dữ liệu, bảng thông tin đăng nhập
CREATE TABLE `users` ( `user_id` int(12) NOT NULL, `name` varchar(50) DEFAULT NULL, `user_name` varchar(50) NOT NULL, `email` varchar(255) NOT NULL, `password` varchar(255) NOT NULL, `status` int(1) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; // Indexes for table `users` ALTER TABLE `users` ADD PRIMARY KEY (`user_id`), ADD UNIQUE KEY `email` (`email`); // AUTO_INCREMENT for table `users` ALTER TABLE `users` MODIFY `user_id` int(12) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=1;
Bước 2: Kết nối đến cơ sở dữ liệu
Để cấu hình đến cơ sở dữ liệu bạn cần thay đổi thông số trong file:application/config/database.php
Ta sẽ chỉ cần để ý đến một số dòng sau trong file:
Thêm dòng code sau vào trong file application/config/routes.php
$route['login'] = 'users/login';
Bước 4: Cập nhật autoload file
Truy cập application/config/autoload.php để cấu hình thư viện
// load libraries $autoload['libraries'] = array('database','session'); // load helper $autoload['helper'] = array('url');
Bước 5: Tạo Model
Tạo file Users_model.php trong thư mục application/models
<?php /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * Description of Users Model * * @author Team TechArise * * @email [email protected] */ defined('BASEPATH') OR exit('No direct script access allowed'); class Users_model extends CI_Model { // declare private variable private $_userID; private $_name; private $_userName; private $_email; private $_password; private $_status; public function setUserID($userID) { $this->_userID = $userID; } public function setEmail($email) { $this->_email = $email; } public function setPassword($password) { $this->_password = $password; } public function getUserInfo() { $this->db->select(array('u.user_id', 'u.name', 'u.email')); $this->db->from('user as u'); $this->db->where('u.user_id', $this->_userID); $query = $this->db->get(); return $query->row_array(); } function login() { $this -> db -> select('user_id, name, email'); $this -> db -> from('user'); $this -> db -> where('email', $this->_email); $this -> db -> where('password', $this->_password); $this -> db -> limit(1); $query = $this -> db -> get(); if($query -> num_rows() == 1) { return $query->result(); } else { return false; } } } ?>
Bước 6: Tạo Control
Tạo file Users.php trong “application/controllers
<?php defined('BASEPATH') OR exit('No direct script access allowed'); class Users extends CI_Controller { /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * Description of Users Controller * * @author Team TechArise * * @email [email protected] */ public function __construct() { parent::__construct(); $this->load->model('Users_model', 'user'); } // Dashboard public function index() { if ($this->session->userdata('is_authenticated') == FALSE) { redirect('users/login'); // the user is not logged in, redirect them! } else { $data['title'] = 'Dashboard - Tech Arise'; $data['metaDescription'] = 'Dashboard'; $data['metaKeywords'] = 'Dashboard'; $this->user->setUserID($this->session->userdata('user_id')); $data['userInfo'] = $this->user->getUserInfo(); $this->load->view('users/dashboard', $data); } } // Login public function login() { $data['title'] = 'Login - Tech Arise'; $data['metaDescription'] = 'Login'; $data['metaKeywords'] = 'Login'; $this->load->view('users/login', $data); } // Login Action function doLogin() { // Check form validation $this->load->library('form_validation'); $this->form_validation->set_rules('email', 'Your Email', 'trim|required|valid_email'); $this->form_validation->set_rules('password', 'Password', 'trim|required'); if($this->form_validation->run() == FALSE) { //Field validation failed. User redirected to login page $this->load->view('users/login'); } else { $sessArray = array(); //Field validation succeeded. Validate against database $email = $this->input->post('email'); $password = $this->input->post('password'); $this->user->setEmail($email); $this->user->setPassword(MD5($password)); //query the database $result = $this->user->login(); if($result) { foreach($result as $row) { $sessArray = array( 'user_id' => $row->user_id, 'name' => $row->name, 'email' => $row->email, 'is_authenticated' => TRUE, ); $this->session->set_userdata($sessArray); } redirect('users'); } else { redirect('users/login?msg=1'); } } } // Logout public function logout() { $this->session->unset_userdata('user_id'); $this->session->unset_userdata('name'); $this->session->unset_userdata('email'); $this->session->unset_userdata('is_authenticated'); $this->session->sess_destroy(); $this->output->set_header("Cache-Control: no-store, no-cache, must-revalidate, no-transform, max-age=0, post-check=0, pre-check=0"); $this->output->set_header("Pragma: no-cache"); redirect('login'); } } ?>
Bước 7:Tạo view file
Tạo file login.php trong thư mục “application/views/users”
<div class="row page-content"> <div class="col-lg-12"> <h2>Login Form</h2> <?php if(validation_errors()) { ?> <div class="alert alert-danger"> <?php echo validation_errors(); ?> </div> <?php } ?> <?php if(!empty($this->input->get('msg')) && $this->input->get('msg') == 1) { ?> <div class="alert alert-danger"> Please Enter Your Valid Information. </div> <?php } ?> <?php echo form_open('users/dologin'); ?> <div class="form-group"> <input type="text" name="email" class="form-control" id="email" placeholder="Email"> </div> <div class="form-group"> <input type="password" name="password" class="form-control" id="password" placeholder="Password"> </div> <div class="form-group pull-right"> <button type="submit" id="login" class="btn btn-primary">Login</button> </div> </div> <?php echo form_close(); ?> </div>